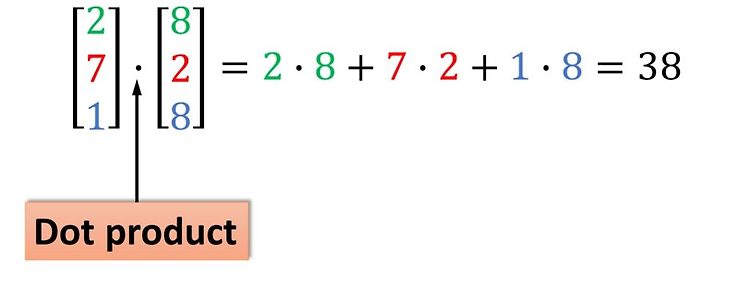
transform() 함수와 accumulate() 함수를 사용해 직접 구현하면 다음과 같다. #include #include #include #include using namespace std; void main() { vector A = {1, 2, 3}; vector B = {4, 5, 6}; transform(A.begin(), A.end(), B.begin(), A.begin(), ::multiplies()); cout
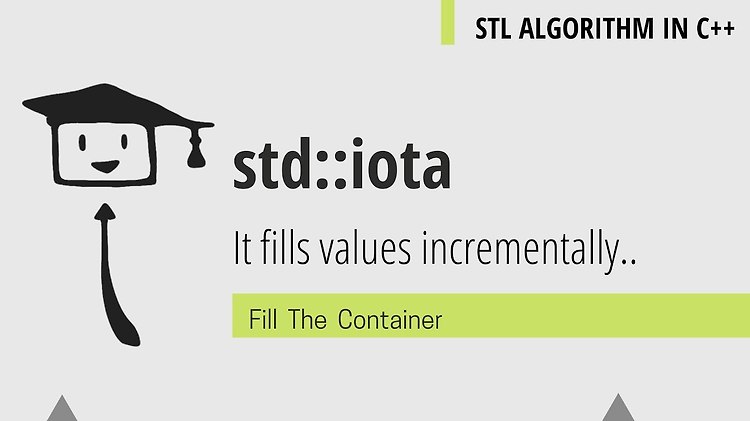
보통은 이렇게 쓴다. #include #include using namespace std; void print(vector v); void main() { vector v(5); for (int i = 0; i < 5; ++i) v[i] = i; print(v); } 출력 0 1 2 3 4 더 쉬운 방법이 있다. #include #include #include #include using namespace std; void print(vector v); void main() { vector v(5); int i = 0; generate(v.begin(), v.end(), [&i]() { return i++; }); print(v); generate(v.begin(), v.end(), [i]() mutabl..
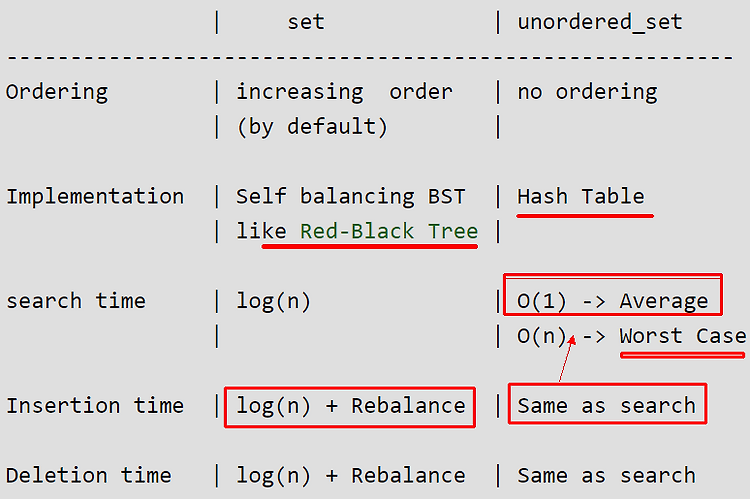
입력을 처리하기 전에 중복이 존재하는지 확인해야 할 때, map 타입으로 bool 값을 따로 저장하지 않아도 된다. set과 unordered_set은 insert() 함수의 반환 값으로 삽입된(혹은 이미 있는) 원소의 반복자와 중복을 확인한 성공 여부를 pair 쌍으로 반환한다. #include #include using namespace std; void main() { set st; pair reuslt = st.insert("LoL!!"); cout
Call by reference of bool types How can I change this piece of code to a working one?! #include void foo( bool &b ) // ERROR in passing argument 1 of ‘void foo(bool&)’ { std::cout
std::replace() 함수를 이용해 컨테이너 범위 사이의 값을 변경할 수 있다. #include #include #include using namespace std; void print(vector v); void main() { vector v1{1, 1, 1, 2, 2, 2, 3, 3, 3, 4, 4, 4}; vector v2; replace(v1.begin(), v1.end(), 1, 0); print(v1); replace_if(v1.begin(), v1.end(), [](int e) { return e == 2; }, 0); print(v1); replace_copy(v1.begin(), v1.end(), back_inserter(v2), 3, 0); print(v2); replace_co..
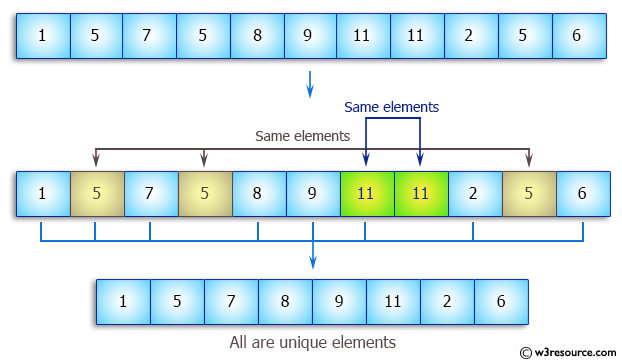
unique가 실행될 때 각 원소는 자신의 양 옆 원소와 비교하여 중복을 제거한다. 그러므로 연속값 제거가 아니라 중복 제거를 원한다면 정렬된 상태에서 사용해야 한다. O(n)에 수행된다. 버블 정렬과 비슷한 방식이며 뒤로 몰아 놓은 중복 원소들의 시작 지점 반복자를 반환한다. #include #include #include using namespace std; void print(vector v); void main() { vector v{5, 4, 1, 1, 1, 3, 2, 2, 7, 6, 6, 8}; cout